How to Create a Chat App in Android
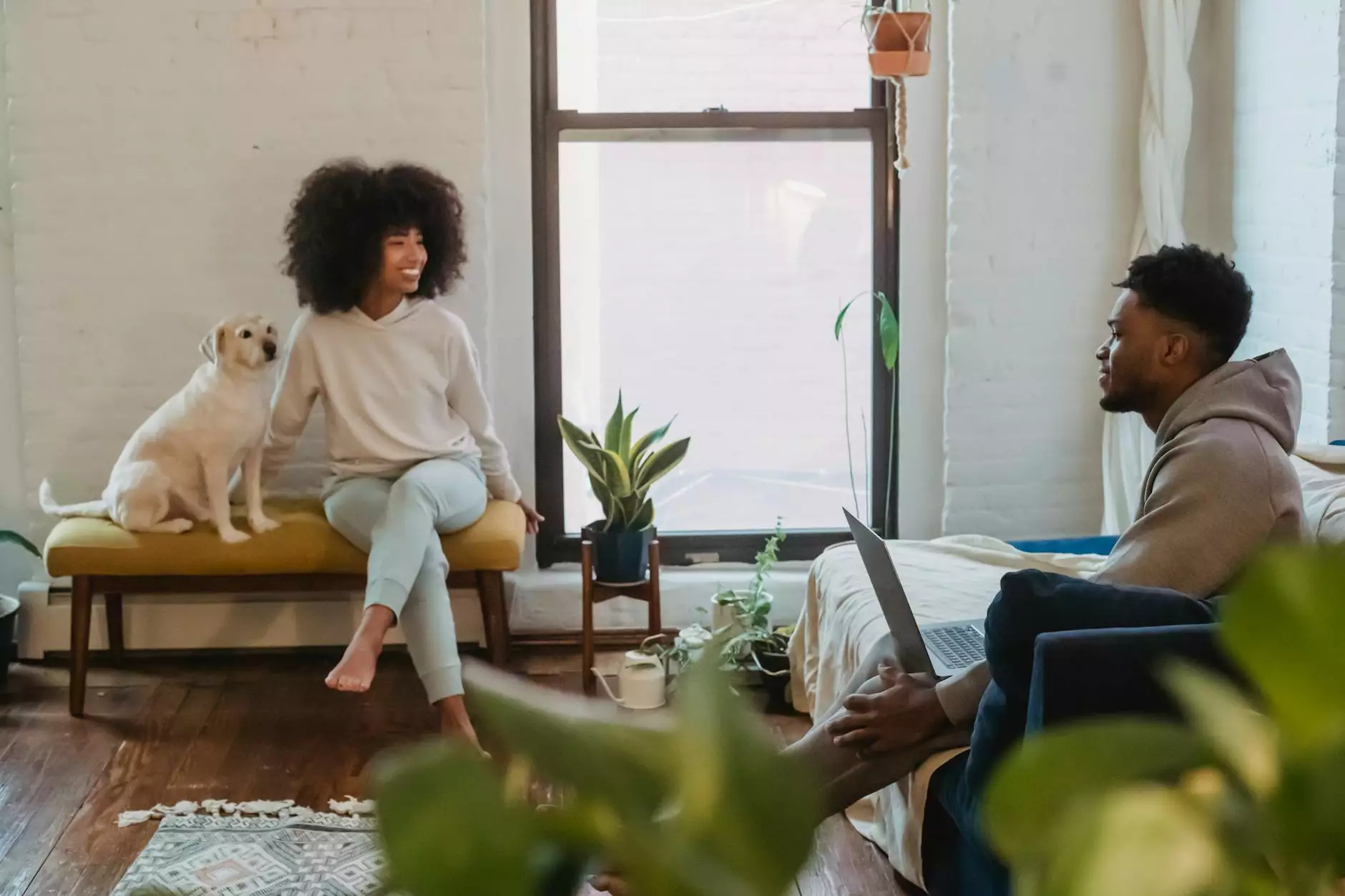
In today’s fast-paced digital world, the need for communication apps has never been greater. Building a chat app in Android opens up opportunities not just for personal projects but also for enhancing business communication. In this comprehensive guide, we will explore the step-by-step process of creating a chat app in Android, ensuring you have all the tools and insights needed to succeed.
Understanding the Essentials of a Chat App
Before diving into the development process, it's crucial to understand what makes a chat app effective. A good chat application should have the following features:
- User Authentication: Secure log-ins through email, phone number, or social media accounts.
- Real-time Messaging: Instant message delivery and receipt notifications.
- Multimedia Sharing: Ability to share images, videos, and files.
- Group Chats: Functionality that allows multiple users to chat simultaneously.
- Push Notifications: Alerts for new messages even when the app is closed.
- User Presence Indicators: Showing online/offline status of users.
Getting Started with Android Development
Creating a chat app in Android begins with setting up your development environment. You will need the following:
- Android Studio: The official IDE for Android development, which offers a robust set of tools.
- Java or Kotlin: Programming languages used to develop Android apps.
- Firebase: A powerful backend platform that simplifies the creation of chat functionalities.
- API Integration: Learn how to connect with third-party APIs for added features.
Setting Up Android Studio
Your first step is to download and install Android Studio. Once installed, create a new project and choose the "Empty Activity" template. This will provide a clean slate for your app development.
Exploring Firebase for Real-Time Database
Firebase is crucial for real-time communication in your chat app. Follow these steps to set it up:
- Create a Firebase project in the Firebase console.
- Add your Android app to the project.
- Download the google-services.json file and place it in your project.
- Add required Firebase dependencies to your app's build.gradle file.
Integrating Firebase allows you to leverage features like user authentication and a real-time database that can store and retrieve messages instantly.
Designing the User Interface
A user-friendly interface plays a significant role in the success of a chat app. Here’s how to design a simple yet effective UI:
Building the Layout
Utilize XML to create the layout files for your chat app. Your main activity layout should include:
- EditText: For inputting messages.
- Button: For sending messages.
- RecyclerView: To display a list of messages.
Here is a sample XML layout snippet:
Implementing User Authentication
Authentication is key for secure user access. With Firebase Authentication, you can implement this feature effortlessly:
- Enable email and password authentication from the Firebase console.
- Use the FirebaseAuth class to register users.
- Implement a login function to allow users to log in.
Here’s a code snippet that demonstrates user registration:
FirebaseAuth mAuth = FirebaseAuth.getInstance(); mAuth.createUserWithEmailAndPassword(email, password) .addOnCompleteListener(this, task -> { if (task.isSuccessful()) { // User registered successfully } else { // Registration failed } });Creating Real-Time Messaging Features
Once authentication is set, you can start with the real-time messaging functionality. Here's how:
Setting Up the Database Structure
In Firebase, structure your database to store messages between users. A sample structure could look like this:
{ "chats": { "chatID": { "messages": { "messageID": { "sender": "userID", "text": "Hello, World!", "timestamp": "timestamp" } } } } }Sending Messages
Once your database is set up, create a function to send messages:
private void sendMessage(String message) { String chatID = "uniqueChatID"; // Replace with your logic to get chat ID DatabaseReference messageRef = FirebaseDatabase.getInstance().getReference("chats").child(chatID).child("messages").push(); messageRef.setValue(new Message(currentUserId, message, ServerValue.TIMESTAMP)); }Receiving Messages
To receive messages in real-time, utilize a Firebase listener:
DatabaseReference messageRef = FirebaseDatabase.getInstance().getReference("chats").child(chatID).child("messages"); messageRef.addChildEventListener(new ChildEventListener() { @Override public void onChildAdded(DataSnapshot dataSnapshot, String s) { // Process the new message } // Implement other required methods });Enhancing User Experience with Notifications
Push notifications keep users engaged by notifying them about new messages even when the app is not open. To implement notifications:
- Integrate Firebase Cloud Messaging (FCM) in your project.
- Send notifications from your backend whenever a new message is sent.
- Implement the FCM receiver in your app to handle incoming notifications.
Testing Your Chat App
Before launching, thorough testing is critical to ensure functionality. Here are some testing strategies:
- Unit Testing: Test individual components, such as message sending and receiving.
- User Acceptance Testing: Have real users test the app to gather feedback on usability.
- Beta Testing: Release the app to a small group of users to identify any remaining bugs.
Launching Your Chat App
After rigorous testing, you're ready to launch your app. Consider the following steps for successful deployment:
- App Store Guidelines: Ensure your app meets all guidelines for the Google Play Store.
- Marketing Strategy: Develop a strategy to promote your app to potential users.
- Monitor Analytics: Use Firebase Analytics to track user engagement and modify your app based on feedback.
Conclusion
Creating a chat app in Android is a rewarding venture that can lead to new business opportunities. By leveraging platforms like Firebase, focusing on user experience, and rigorously testing your application, you can build a robust communications tool that meets user needs. As you embark on this journey, remember to focus on continuous improvement based on user feedback and engagement analytics.
Ultimately, the key to a successful chat app lies in its ability to engage users through reliable, fast, and user-friendly communication. Keep innovating, and your app could become a go-to communication solution for many!
how to create a chat app in android